반응형
React Native Modal Functional Component Hooks
Modal은 특정 이벤트가 실행되었을 때, 화면 상단에 표시되는 창으로, 화면 전체를 다 가리지 않고 일부만 가리는 것이라고 생각하시면 됩니다.
사용자의 응답(reponse)를 요구하며 확인, 예/아니오 등의 응답을 요구합니다.
응답이 있기 전까지 화면에서 사라지지 않으며 응답을 받으면 사라지는 동작입니다.
Modal은 React Native에 기본으로 포함되어 있는 기능이기도 합니다만,
별도로 react-native-modal을 설치해서도 사용을 합니다.
저는 React Native에 기본으로 내장되어 있는 modal을 가지고 보여드리겠습니다.
두 파일로 분활하여 구현하였습니다.
AppModalContainer.js
import React from 'react';
import { Modal, SafeAreaView, StyleSheet } from 'react-native';
const AppModalContainer = ({
children,
}) => {
return (
<Modal
animationType="fade"
>
<SafeAreaView style={styles.container}>
{children}
</SafeAreaView>
</Modal>
)
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'rgba(10, 19, 33, 0.56)',
},
})
export default AppModalContainer;
여기서는 Modal과 SafeAreaView만 포함해주었구요 실제 Modal은 children으로 들어오는 방식입니다.
AppModal.js
import React from 'react';
import {
View,
StyleSheet,
TouchableOpacity,
Text,
} from 'react-native';
import { colors, height, width } from '../../config/globalStyles';
const AppModal = ({
children,
negativeButton,
positiveButton,
negativeButtonPress,
positiveButtonPress,
}) => {
return (
<View style={styles.container}>
<View style={styles.contentContainer}>{children}</View>
<View style={styles.buttonContainer}>
{negativeButton &&
<TouchableOpacity
style={styles.negativeButton}
onPress={negativeButtonPress}
>
<Text style={styles.text}>
{negativeButton}
</Text>
</TouchableOpacity>
}
<TouchableOpacity
style={styles.positiveButton}
onPress={positiveButtonPress}
>
<Text style={styles.text}>
{positiveButton}
</Text>
</TouchableOpacity>
</View>
</View>
);
};
export default AppModal;
const styles = StyleSheet.create({
container: {
width: width * 280,
paddingTop: height * 20,
alignItems: 'center',
borderRadius: 20,
backgroundColor: 'white',
},
contentContainer: {
paddingBottom: height * 20,
},
buttonContainer: {
flexDirection: 'row',
},
positiveButton: {
flex: 1,
padding: 10,
alignItems: 'center',
},
negativeButton: {
flex: 1,
padding: 10,
alignItems: 'center',
},
text: {
fontSize: 16,
}
});
기본적인 확인과 취소가 있는 Modal을 만들었습니다.
취소(negativeButton)이 없다면 확인 버튼만 있게 만들었습니다.
내용으로 들어올 부분은 children으로 들어오는 방식입니다.
이렇게 하여 좀 더 유연하게 내용이 바뀌어도 동작할 수 있게 만들었습니다.
실행은 다음과 같이 하였습니다.
import React from 'react';
import { storiesOf } from '@storybook/react-native';
import { BufferView } from '../Decorator/Decorators';
import AppModal from '../components/Modal/AppModal';
import AppModalContainer from '../components/Modal/AppModalContainer';
import { Text } from 'react-native';
storiesOf('Modal', module)
.addDecorator(BufferView)
.add('default', () => (
<AppModalContainer>
<AppModal
positiveButton="확인"
negativeButton="취소"
>
<Text> App Modal Test </Text>
</AppModal>
</AppModalContainer>
));
저는 storybook을 사용하였기에 조금 형식이 다른데요,
<AppModalConatiner>태그 내의 내용만 보시면 됩니다!
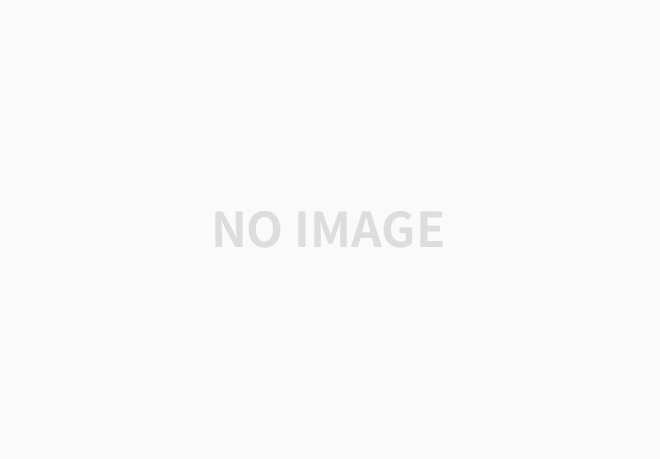
반응형
'웹, 앱 개발 > RN React-Native' 카테고리의 다른 글
[React Native] react-native-stopwatch-timer (0) | 2021.01.25 |
---|---|
[React Native] Header - Functional Component Hooks (0) | 2021.01.24 |
[React Native] Text - Functional Component Hooks (0) | 2021.01.23 |
[React Native] Navigation Option no Header 헤더 없애기, 제거 (0) | 2021.01.23 |
[React Native] GlobalStyles 전역(공통) 스타일 (0) | 2021.01.23 |